๐ขย AI Code Assistant
You can use ChatGPT for code generation, generating test cases, commenting, reviewing, and vulnerability detection.
Code Generationโ
We can have ChatGPT automatically generate a Python code for the quicksort algorithm.
Simple code generation is an easy task for ChatGPT.
Test Casesโ
Test cases are sourced from StuGRua.
With a clearly defined function to be tested, unit tests can be generated by ChatGPT.
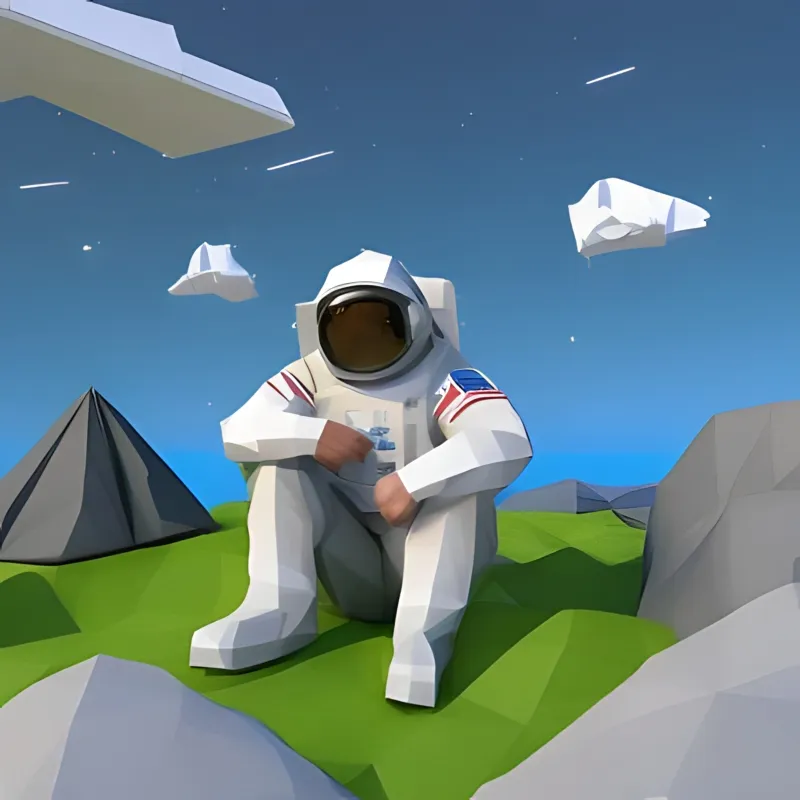
Prompt
Write unit tests for the CompressPicture function below, covering all logic. Use the require package for assertions. Output the test cases in the form of testCases.
// CompressPicture the picture by resizing it to the specified width. func CompressPicture(picData []byte, width uint) ([]byte, error) { // Decode the input image. oldBuffer := bytes.NewBuffer(picData) inputImg, _, err := image.Decode(oldBuffer) if (err != nil) { return nil, err } // Resize the image to the specified width. outputImg := resize.Resize(width, 0, inputImg, resize.Lanczos3) // Encode the output image as PNG and write it to a buffer. newBuffer := new(bytes.Buffer) err = png.Encode(newBuffer, outputImg) if (err != nil) { return nil, err } // Return the compressed image as a byte slice. return newBuffer.Bytes(), nil }
func TestCompressPicture(t *testing.T) {
// Setup test data
imgData, err := os.ReadFile("test-image.png")
if (err != nil) {
t.Fatalf("failed to read test image file: %v", err)
}
testCases := []struct {
name string
width uint
}{
{"compress with a valid width", 500},
{"compress with an invalid width", 0},
}
for _, tc := range testCases {
t.Run(tc.name, func(t *testing.T) {
// Invoke the function being tested.
compressedData, err := CompressPicture(imgData, tc.width)
// Check the result.
if (err != nil) {
t.Fatalf("unexpected error: %v", err)
}
if (len(compressedData) == 0) {
t.Fatalf("compressed data is empty")
}
create, err := os.Create("test-image-compressed.png")
if (err != nil) {
return
}
defer create.Close()
_, err = create.Write(compressedData)
})
}
}
ChatGPT's single conversation length is limited, so try to generate unit tests for one function at a time.
Code Comments & Format Optimizationโ
ChatGPT can read code, understand the inputs, outputs, functions, and purposes of the code, and then generate corresponding API documentation and comments.
Please add line comments to this Python code and reformat it to make it easier to read:
import openai
openai.api_key = "YOUR_API_KEY"
def generate_response(prompt):
response = openai.Completion.create(engine="davinci",prompt=prompt,temperature=0.5,max_tokens=1024,n=1,stop=None)
return response.choices[0].text.strip()
while True:
prompt = input("่ฏท่พๅ
ฅไฝ ็้ฎ้ข๏ผ")
response = generate_response(prompt)
print("็ญๆก๏ผ", response)
ChatGPT provided explanations for each line and converted overly long statements into a more readable format.
import openai # Import the openai module
# Set the API key for the openai module
openai.api_key = "YOUR_API_KEY" # Replace with your actual OpenAI API key
# Define a function for generating a response from OpenAI's language model given a prompt
def generate_response(prompt):
# Create an OpenAI completion with the specified parameters
response = openai.Completion.create(
engine="davinci", # Use the 'davinci' language model
prompt=prompt, # Use the provided prompt
temperature=0.5, # Use a temperature of 0.5, which means the model will generate diverse responses
max_tokens=1024, # Generate up to 1024 tokens of output
n=1, # Generate a single response
stop=None # Do not use a stop sequence to end the generated text
)
# Extract the generated text from the response and return it
return response.choices[0].text.strip()
# Continuously prompt the user for questions and generate responses using the generate_response function
while True:
prompt = input("Please enter your question: ") # Prompt the user for a question
response = generate_response(prompt) # Generate a response to the question using the generate_response function
print("Answer:", response) # Print the generated response
Code debuggingโ
Through the previous steps, you have learned how to write the correct code with test cases.
In daily development, in addition to writing code, we will also encounter two tricky development steps. One is to solve the errors after running the existing code; the other is how to reproduce the processing logic already supported by other programming languages in the current programming language?
Let's solve the first problem first. Generally speaking, run after writing the code. If there is an error in the code, the interpreter will prompt the error message. According to the prompt information, the error can usually be found and modified quickly. But sometimes the error message is not so clear. When you check it several times and still can't find the problem, it will be more troublesome. ChatGPT can combine existing error messages to help us analyze potential problems in the code.
Here I selected an example of python and SQL database connection error on stack overflow: Error while trying to connect to sql server with python using pyodbc
ChatGPT played the role of a python expert:
You are a python expert who can pinpoint errors in your code through error messages and provide easy-to-understand solutions.
error```
Traceback (most recent call last):
File "[test.py](http://test.py/)", line 7, in <module>
conn = pyodbc.connect('DRIVER={ODBC Driver 17 for SQL
Server};SERVER='+server+';DATABASE='+database+';UID='+username+';PWD='+ password)
pyodbc.InterfaceError: ('28000', "[28000] [Microsoft][ODBC Driver 17 for SQL Server][SQL
Server]Login failed for user 'SA'. (18456) (SQLDriverConnect); [28000] [Microsoft][ODBC
Driver 17 for SQL Server][SQL Server]Login failed for user 'SA'. (18456)")
code```
import pyodbc
server = 'SERVER'
database = 'DBNAME'
username = 'SA'
password = 'SQLPWD'
conn = pyodbc.connect('DRIVER={ODBC Driver 17 for SQL Server};SERVER='+server+';DATABASE='+database+';UID='+username+';PWD='+ password)
Please analyse "code" for possible causes, solutions and the correct code depending on the "errorโ.
```python
### GPT Output
The error message indicates that the login for the SQL Server failed for the user 'SA'. There could be several reasons for this error, but one possible reason is that the provided password is incorrect or the 'SA' account is disabled. Here are some steps to troubleshoot and fix the error:
Verify the username and password: Double-check that the provided username and password are correct. You can try logging in to the SQL Server using these credentials to ensure that they are valid.
Check if the 'SA' account is disabled: If the 'SA' account is disabled, you will not be able to log in to the SQL Server using this account. You can check the account status in SQL Server Management Studio or by running a query on the server:
```python
SELECT name, is_disabled
FROM sys.sql_logins
WHERE name = 'SA'
If the 'is_disabled' column value is 1, then the account is disabled. To enable the account, you can run the following query:
ALTER LOGIN SA WITH PASSWORD = '<new_password>', CHECK_POLICY = OFF, CHECK_EXPIRATION = OFF;
Note that it's not recommended to use the 'SA' account for regular database operations as it has elevated privileges.
Check the SQL Server authentication mode: If the SQL Server authentication mode is set to 'Windows Authentication mode only', you will not be able to log in using SQL Server authentication. You can check the authentication mode in SQL Server Management Studio by right-clicking on the server instance, selecting Properties, and navigating to the Security tab.
The solution given by ChatGPT is:
- Verify the user name and password
- Check whether the "SA" account is disabled
- Check the authentication mode of SQL Server
The most popular replies on the web page are:
- SA is banned from access
- Error in server authentication selection
- The SQL server does not have open remote access
Through comparison, we can easily get the feasibility of ChatGPT in code debugging.
Code translationโ
Another very useful function provided by ChatGPT is the ability to port programs from one language to another.
Here I found a common algorithm problem in Leetcode [the longest palindromic substring] (https://leetcode.cn/problems/longest-palindromic-substring/) C++ Implementation, converted into Python language.
The longest palindrome substring title: Give you a string s and find the longest palindrome substring in s. If the reverse order of the string is the same as the original string, the string is called a palindy string.
User Inputโ
You are an expert program developer with extensive experience in writing C++ and Python. Please convert this C++ program to Python:
class Solution {
public:
string longestPalindrome(string s) {
int len=s.size();
if(len==0||len==1)
return s;
int start=0;
int max=1;
vector<vector<int>> dp(len,vector<int>(len));
for(int i=0;i<len;i++)
{
dp[i][i]=1;
if(i<len-1&&s[i]==s[i+1])
{
dp[i][i+1]=1;
max=2;
start=i;
}
}
for(int l=3;l<=len;l++)
{
for(int i=0;i+l-1<len;i++)
{
int j=l+i-1;
if(s[i]==s[j]&&dp[i+1][j-1]==1)
{
dp[i][j]=1;
start=i;
max=l;
}
}
}
return s.substr(start,max);
}
};
The code output of ChatGPT is as follows:
class Solution:
def longestPalindrome(self, s: str) -> str:
n = len(s)
if n == 0 or n == 1:
return s
start = 0
max_len = 1
dp = [[0] * n for _ in range(n)]
for i in range(n):
dp[i][i] = 1
if i < n - 1 and s[i] == s[i+1]:
dp[i][i+1] = 1
start = i
max_len = 2
for l in range(3, n+1):
for i in range(n-l+1):
j = i + l - 1
if s[i] == s[j] and dp[i+1][j-1] == 1:
dp[i][j] = 1
start = i
max_len = l
return s[start:start+max_len]
The code successfully passed the test:
Here I recommend [Cursor.so] (https://www.cursor.so/), which is an integrated GPT-4, which can be accessed directly in China. It is an excellent and powerful free code generator that can help you quickly write, edit and discuss. Code. It supports multiple programming languages, such as Python, Java, C#, JavaScript, etc., and can automatically generate code fragments according to your input and requirements.